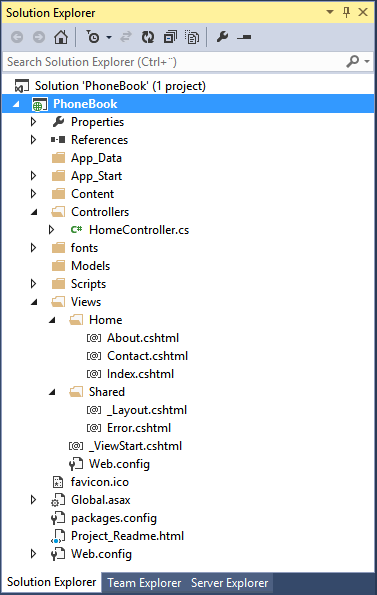
I have expanded a few of the folders, because they will be of first interest to us. There are other very important folders, but they will not be discussed here, since they are only altered when we do more advanced things, and Visual Studio has already set them up properly for out little project.
The Controllers folder contains one file, the HomeController.cs. It is the one responsible for the first page you just saw when running the project, as well as the pages 'About' and 'Contact'. You could see three links 'Home', 'About' and 'Contact' in the header bar of the page when you run the program.
I have also expanded the Views folder. As you can see, it has a sub-folder for the three views, or pages, that is associated with the Home page in the HomeController. There is always, or should always be, a folder with a <name> under the Views folder corresponding to a <name>Controller.cs file in the Controllers folder. You will see why when we continue the discussion below.
You can also see a Shared sub-folder under the Views folder. This is a very convenient way to collect things that are common to all pages, like scripts, cascading style sheets, header, footer, menu and other common things you might need.
It is depending on the file _ViewStart.cshtml, but I will not say more about that one than that it is involved in selecting the proper page to show, when the controller calls a function View() as we will see later.
The file _Layout.cshtml is the one containing all common stuff, like the header and footer, scripts, css's etc. However, the files are mostly not loaded the common HTML way, but rather using Razor and the framework provided by Visual Studio. If you are curious, take a look in the file App_Start\BundleConfig.cs. If you later decide to put in your own css's and/or script files, do it like it is done there. Maybe I will go into that later.
There is a file Error.cshtml that is used if something goes wrong, and you may change it to your liking.
There is also a Model folder for the models, but there are no models yet, so it is empty.
Model View Controller
The idea of MVC is to split the work into areas that are responsible for different things. Such a division makes it easier to work with the code, and to understand it.Just think of it. You are looking at a web page, and what do you see? You see some content presented on your screen. This implies that there is some content, that the content has been prepared some way, and that it has been presented on your screen, in your browser.
M is the model. It describes the content. It is in fact one or more classes describing the data you are watching in your browser.
V is the view. It is the generated html code that makes up what you see in your browser.
C is the controller. It controls what page your see, what you do with the data, and what data you see on the page.
MVC uses restless linking
Now, what is 'restless'? Well, to be frank, I did not quite understand that term. But I know the technicalities behind it.As you might know, a web form can send data to a web page in two ways, via GET and via POST.
This is all about the HTML protocol. Basically it is a question about how to send the data, together with the link or as a separate bunch of data.
You do not need to understand the protocol to be able to use the two methods. It is simply a matter of setting the 'method' attribute of the web form. Here is an example of a POST form:
<form method="post">
<input type="text" />
<input type="submit" />
</form>
Here is an example of a GET form:
<form method="get">
<input type="text" />
<input type="submit" />
</form>
Rather than using a form it is possible to send 'GET' data from the browsers address field:
http://www.avira.com/en/avira-free-antivirus?dlsrc=homepage#start-download
The link above does the same as a GET form at avira.com/en/avira-free-antivirus:
<form method="get">
<input type="text" id="dlsrc" />
<input type="submit" />
</form>
...if the text input has the value homepage#start-download entered or:
<form method="get">
<input type="hidden" id="dlsrc" value="homepage#start-download"/>
<input type="submit" />
</form>
But in the actual case it is just a link, like the one I started out with. Thing is that you could use a GET form to produce the same URL. Did you notice that the id attribute of the hidden input is the part between '?' and '=' in the link, while the value is the part after the '='? If you study some GET links on the Internet, you will most probably also see that there are ampers and marks as well, and that there is always only one question mark. The queston mark marks the start of the id/value pairs listed in the URL, while the ampers and divides the id/value pairs so that the web server knows when a new id/value pair starts. And, of course, the equal sign splits the id/value pair into id and value.
The 'restless' part
The restless linking is partly like GET, but it does not use a question mark to show where the arguments starts. In fact, the first argument is always an id, if present, but it looks like a part of the URL path. Why? Because it does not start with a question mark, but with a slash.Try this: www.mrmartin.eu/Razor/MVC/Hi!.
If you avoid or replace characters that are not allowed in a URL, you can type anything after the trailing slash. One thing not allowed are blanks, but you can use a percent sign to enter the ASCII code instead. The ASCII code for a space is 20. Try entering 'Hello%20world!' after www.mrmartin.eu/Razor/MVC and see what happens (do not include the qoutation marks).
It is also using the POST protocol, but we will take a look at that a little later.
The Controller controls the View
So, how did I do that?The controller controls what you see in the view. If you look at the URL again you will see two parts after www.mrmartin.eu separated by slashes. Those parts tells the web server which controller to use, and which function in the controller to call.
The controller is named like the first part, but with the text 'Controller' added to it. In our case for the page you are looking at, the controller is named 'RazorController' and the function is named 'MVC':
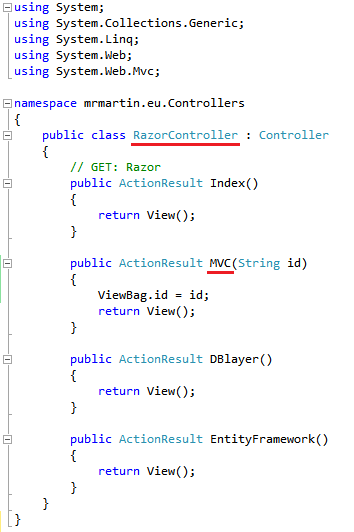
We will discuss more about the controller in a minute, but note that the function MVC takes an argument id of type String. The only thing I do here is to put the id into something called a ViewBag. The ViewBag is an object that passes data to the view.
As you can see, all functions returns a call to the View() function. We will discuss that too in a minute, but for now it is enough to know that it causes our view to be compiled and sent to your browser.
I have put in a peice of code that executes when the html code, that was created when the view was compiled, has been loaded into your browser. Look at the part under the comment :
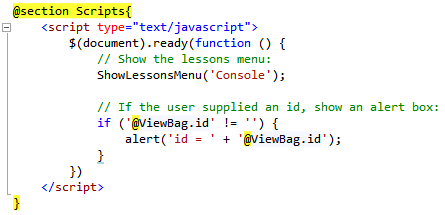
When the view is compiled, the text @ViewBag.id will be replaced with the value we put into it. So, if we did not put anything into it, the code would look like this:

Since '' != '' is false, no alert box will pop-up.
If we typed 'Hello%20world!' in the URL, the text 'Hello world!' would be in ViewBag.id, and @ViewBag.id would be replaced by 'Hello world!' (except the qoutation marks, that is why you have to put it into the code surrounding @ViewBag.id)

Since 'Hello world!' != '' is true, the alert box will pop-up with the content 'id = Hello world!'.
Most browsers have a function to show the html code sent to your browser. Try right-click and select 'View source' or something similar, and see for yourself what the compiled html code looks like.
More about the controller
Have a look at the controller again: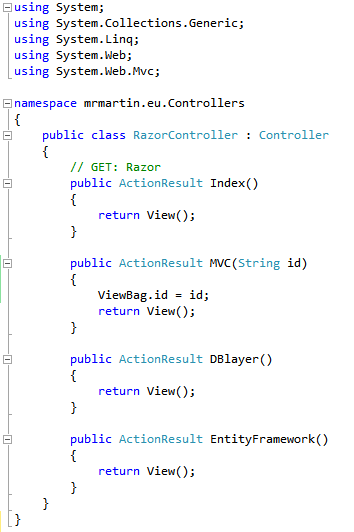
The methods
This is the actual controller for the page you are viewing right now. Note that there are four methods, Index(), MVC(), DBlayer() and EntityFramework(). I have finished the first two when writing this. Actually, I'm working on the second one. The first one, Index() is special in that sense that it is the default method to call if no method name is present in the URL. Try the URL's http://www.mrmartin.eu/Razor and http://www.mrmartin.eu/Razor/Index. They both go to the start page of the ASP.Net web program with Razor lesson. The other functions are only called when specified in the URL, like this one you are reading now (MVC).The ViewBag
The ViewBag is an object that can be assigned values by your choice. You can add id as I did, but the name is not important, just has to be unique. You can name it GrandPa if you like.Any type is allowed, but be aware, code completion (intelli-sense) can not help you, and compiling does not find any errors, but you will get ugly error messages at run-time if you misspell a name or make some other error.
The View() function
The last thing is the View() function. It is part of the ASP.Net API and by calling it you get the view compiled. The result is of type ActionResult. I do not know more about it, but that is enough to make it work. We will later see other ways to end a function when we store data in a database, and wish to re-route to some other page.To summarize, the controller is the one that looks at the last part(s) of the URL to select one of its methods, and accepts an argument by the name id of some type, if present. It is also the controller that prepares any data for the view to show, and that calls View() in order to have the view shown.
The Model
The folder Models can contain one or more class files that describes data. It may be a class corresponding to a database table or view, or some field from a database table or view. The class can describe whatever you need.A model is a handy way to pass a class to the view, and for the view to use in Razor code. We will learn more bout the Model when we start using databases.
The view
The view is the part that you see on your screen. When presented to your browser, it is plain HTML code, but on the server side it is much more.Razor code
We are going to use Razor code in this course. Razor is what makes the page dynamic rather than static. So, what is the difference?A static HTML page contains only HTML code. Well, ok, it can contain script code as well. It is static in the sense that it is sent as is to your browser without any processing on the web server. You see a static page that will not change. If you have any scripting, like the one you can see on mrmartin.eu home page, you can actually have it look dynamic. But it is still static, because it will behave the same way everytime. Even if you have some scripting in it. (Ok, there are random generators, but still, it will only produce random numbers, not obtain new data.)
The main thing is that it will not change by requesting new data from the server. Well, ok, there are ways to do that too, and maybe I will take that up later.
So, if we can disregard those types of dynamic behaviour, let us define the opposit of static pages now: A dynamic page is a page that is processed by some program integrated into the web server (called ISAPI filter for the geek) in order to produce a page that is depending on some data, and thus is not the same everytime it is called.
A very simple example is a page that has a time-stamp, like the one below:
23/07/2025 5:07:29 am
The controller has been updated with one line:
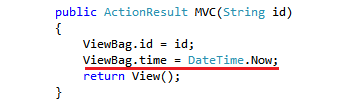
...and in the view code, I have added @ViewBag.time.
If you reload the page, you will see that the time updates. It will be obtained from the computer the web server is running on, and brand new data will be supplied to your browser. Try it! Press F5 and see how the time changes.
The code @ViewBag.time that I have embedded into the HTML code is the actual Razor code. When the page is called, the web server will parse it before sending it, and it will execute any code that starts with an @ and ends by entering any html tag. If you need to end it without typing a tag, you can end it using @: as an "end Razor code tag". You can also use curly brackets, like this:
@{
// Some code here:
ViewBag.time;
}
If you look into the project we created first in this course, the view files are all of type .cshtml. That is the type for Razor with C# code, and that is what we will use in this course.
// Some code here:
ViewBag.time;
}
Time-stamp exercise
As a first exercise, insert a time-stamp into that first project on the 'About' page. Add a line in the HomeController's 'About' method that puts the time in the ViewBag like I did above, and put it in the file ...\Views\Home\About.cshtml. Since all is given here, I will not put it in 'Code samples'. I'm sure you will be able to solve it.There is much more to the MVC structure, but it will be more obvious if we have some data, so I think it is time to create a database layer for our project.