JavaScript
Merlin »
<html>
<head>
<script type="text/javascript">
function AlterLabel(Object) {
Object.textContent =
'You clicked me! How dare you?';
}
</script>
</head>
<body>
There is a label here below, I wouldn't dare
click it if I were you!<br />
<label id="MyLabel" onClick="AlterLabel(this);">
Don't click me!
</label>
</body>
</html>
<head>
<script type="text/javascript">
function AlterLabel(Object) {
Object.textContent =
'You clicked me! How dare you?';
}
</script>
</head>
<body>
There is a label here below, I wouldn't dare
click it if I were you!<br />
<label id="MyLabel" onClick="AlterLabel(this);">
Don't click me!
</label>
</body>
</html>
This time I will go into the technical parts. There are two things to discuss here before we go into the JavaScript stuff.
First of all, this is a client-side program, or script to be precise. Java-script. The thing here is that it is client-side. The other type is called server-side scripting or programming.
Second we have the difference between programs and scripts. If you have hade a look into the console course you have seen programs.
Client-side versus server-side
A script or a program that you use over some network, most common the Internet, will run code on two or more computers. The discussion here is about the two endpoints. Your client computer and the computer that serves your request.You are right now looking at mrmartin.eu. You can do that because you have a program in your computer that can request pages from the Internet, in this case a page from mrmartin.eu, and that there is a server on the internet that will respond to your request.
Both computers has programs to do this for you. Your computer has an Internet browser, like Internet Explorer, Google Chrome, Opera or Firefox. The server on the other end of your connection has a program called a Web server, like Windows Internet Information Server, IIS or Apache, a web server that works on both Windows and Linux.
In the internet youth the web server could only do one thing: I could send you text files. With the HTTP protocol, the text files could contain links to other files. Then if you click on a link, your computer would make a new request to the web server to send another text file. The content of those files were static. They were the same each and every time you requested it, unless someone replaced the file on the web server.
Today we have dynamic pages. They are not the same everytime. E.g. this timestamp: 27/06/2025 5:34:06 am changes everytime you request this page. Right now it shows the time for when you opened this page. If you were to refresh the page (F5), the time updates. In this case it is the server that modifies the text in the HTML file for this page, before sending it to you. You will only see a new time if you request the page anew from the server. That is what we call server-side program or script. In this case it is a program, but we will get into that later.
If we on the other hand have a program or script sent over with the HTML file, it may be executed in your browser, unless it is configured to prevent code execution. Most, if not all, browsers has setting for preventing programs to run in the browser. See 'Settings' at the bottom of this page.
Try clicking this label: and see how it changes to show you current time. It is the time your machine says it is. It should be the same time, since the server asks for the ITC time, and the JavaScript asks for the GMT time, and that should be the same time. The display format is a little different as well, but that is due to how the string formatting is done in the different programs.
Just to make sure that you see the difference, I have layed out the different ways to fetch the time here. However, the server side time is now introduced as a link, so you may click on that one as well.
- 27/06/2025 5:34:06 am
Try clicking the two links and observe the difference. The first link causes the brower to fetch the entire page anew. The server updates the time and sends the new version of the page to you.
The second link, however, just updates the time without resending the page. The time is fetched from your local computer, whatever way it knows about the time.
Scripts versus programs
Here's the difference between scripts and programs:- A program is compiled into executable code that the processor can run.
- A script is a file with program instructions in clear text, that is run by a program, a script engine.
So, if you compile, and probably also link, your program into an executable, then it is a program.
If you do not compile your program, but have another program that can read the text written in your program and execute it line by line, then it is not a program. It is a script.
Programs and scripts have limilar syntax, but often differs enough to make it suitable only as a program to compile, or a script to run by another program. Such a program is called a script engine.
How can a brower and a web server run scripts?
It is not the main task for a web server or a browser to run scripts. In fact, early web servers and early browsers could not do that. However, modern web servers and browsers can. The program parts that does that is still not a part of the web server nor the browser. Instead, plug-ins are used. A plug-in is simply a dll file containing the program code to add to the web server or the browser. In order for the web server or the browser to use the program code in the dll file, it must have certain functions that both are aware of.The use of dll files makes it possible to update the plug-in independently of the host program, the web server or browser.
The script engine in the dll is simply a program that understands the sourcecode in the same way as a compiler. The difference lies in that the script engine does not compile the sourcecode into executable code. Instead it interprets the code line-by-line and uses it's own pre-compiled functions to do the work.
So, in the dll we have some executable code, some is for parsing the script and some contains functions that executes whatever your script code needs to have executed.
Let's compare with an example:
A windows program that has a messagebox that displays a text. When you compile your program, it will link in the code necessary for displaying a messagebox, and place a call to the messagebox into your executable.
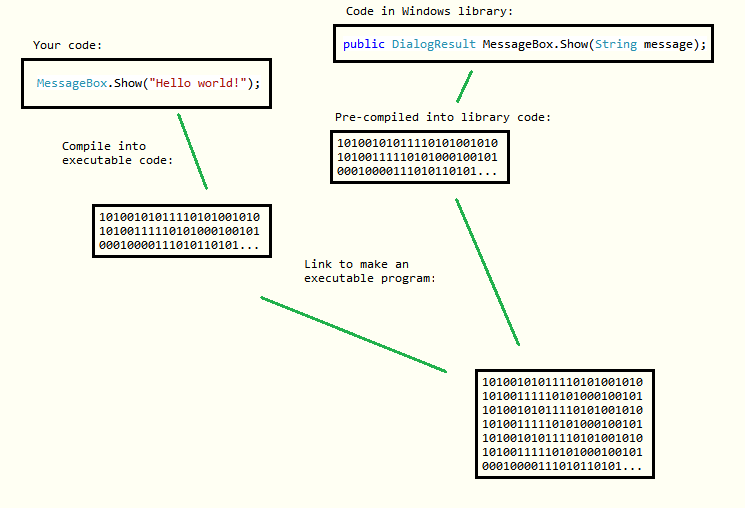
A JavaScript has an alertbox rather than a messagebox. They both do the same thing. When the executable code in the dll containing the script engine for JavaScript reads your code:
alert('Hello world!');
it will call one of its internal functions passing the text 'Hello world!' as an argument. That internal function is already linked to a
function in the operating system, a messagebox in case of Windows, and the message pops up on your screen. See the difference?
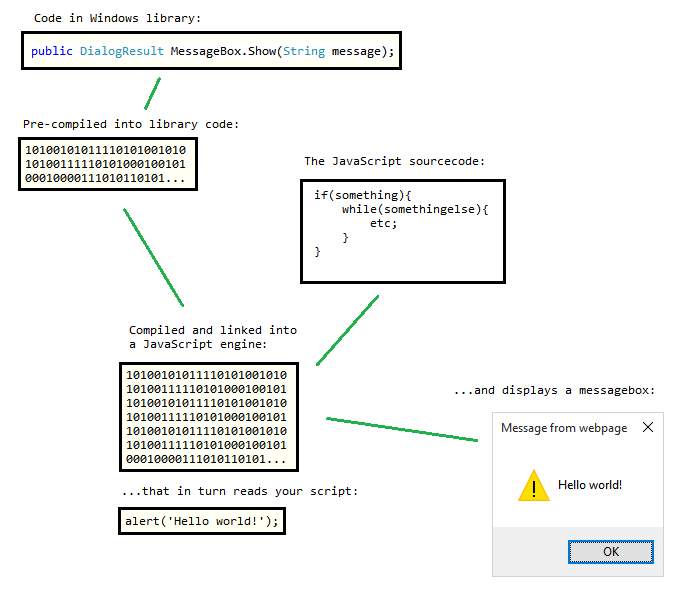
The DOM and the API
The web browser uses a set of objects to represent the different parts of a web page. That is only natural for object oriented programs, OOP. The set of objects is called the DOM, the Document Objects Model. This is a standard, so you should be able to use the same objects in every compatible browser.Here are a few of the objects:
- document - represents the entire web page
- forms - represents a list of all forms in a document
- window - represents the window containing the document
- location - represents the url of the document
Note that DOM is hierarchical, so you can e.g. adress a form by typing
document.forms[0]
in order to address the first form of a page.And here are a few things from the API:
- alert() - Shows a messagebox
- Math - a math library
- getElementById() - finds an html element by its id attribute
- Math.random() - a random number generator in the Math library
That was only a very few examples of what you can find in the DOM and the API. You will have to search the Internet for further documentation, or just ask mr Google when you need something. Also check the 'Tips´n tricks´n other stuff' menu for links.
If you feel up to it, let's make a JavaScript client-side game, Merlin!
Settings
This is how to allow script programs to run in Internet Explorer 10: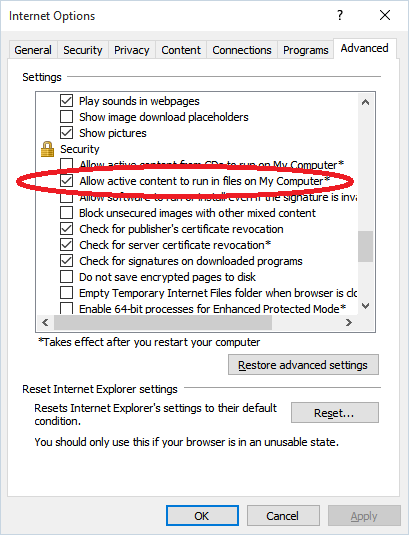
Other browsers has similar settings. If you cannot find it, ask mr Google.