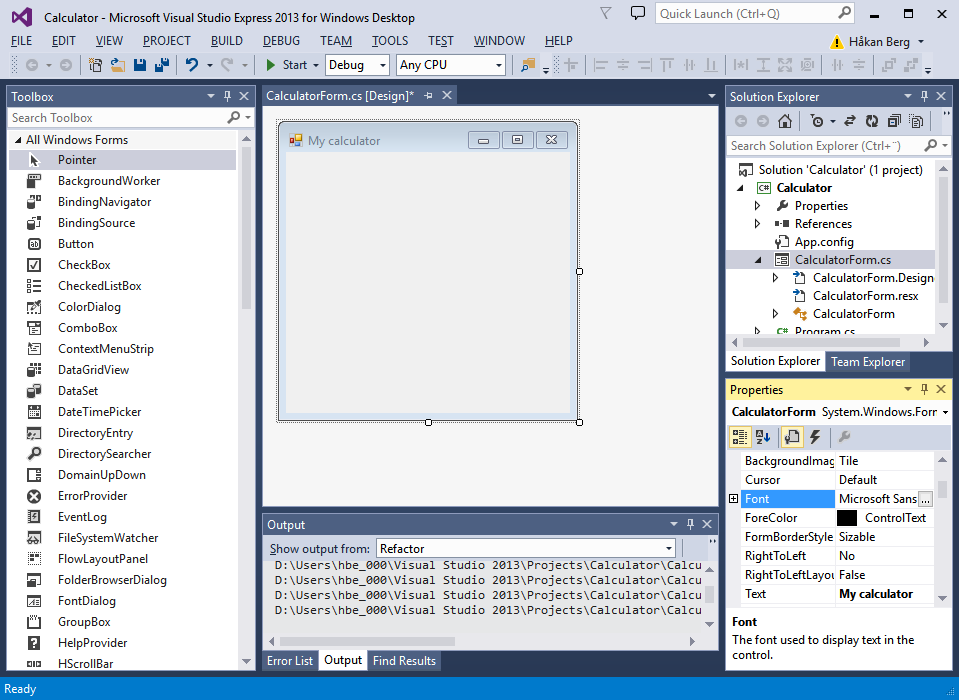
Next, find the Form Properties Font property and increase the font to 16 points:
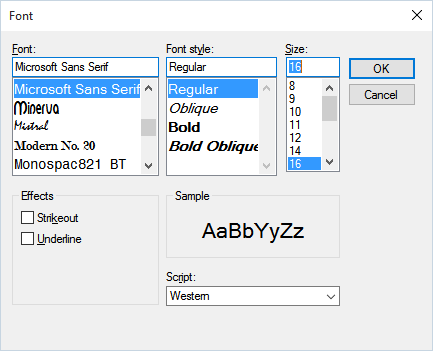
Design the form as shown below. Name the controls in a manner so that you know what controls does what when we come to coding. If you want to use the same name as I have used, look ahead on this page, or check the Code samples. This i how I designed it:
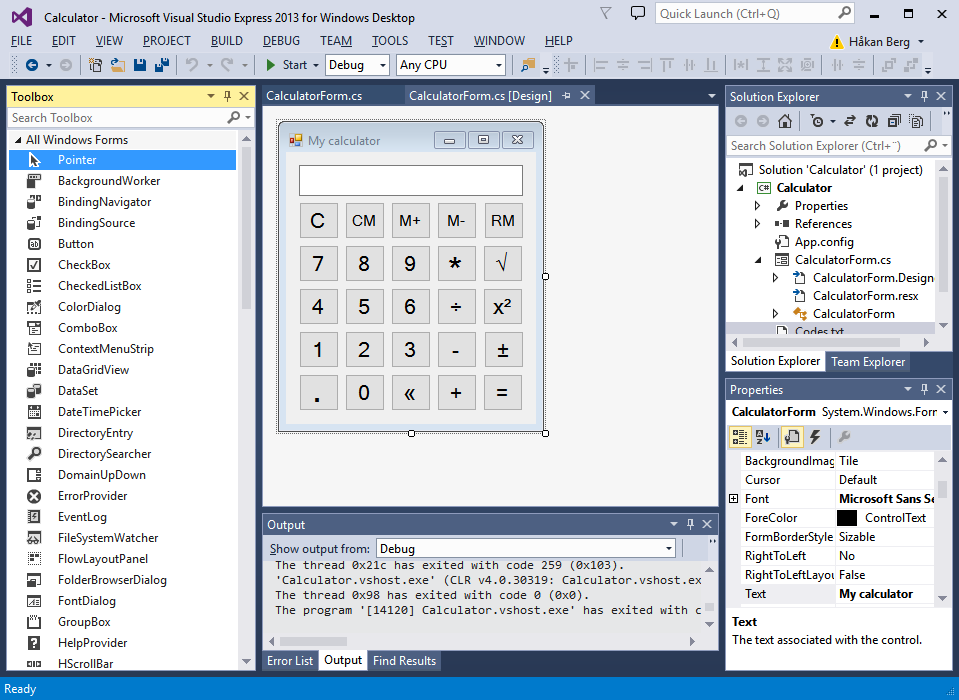
Note that I had to change font size for some buttons in order to be able to show more than one character. It is good practice to document stuff, and I have added a file Readme.txt where I documented the Alt codes that creates the special characters you see in the form.
If you like, you can use image buttons instead. Then you'll have to ask Mr Google on how to do that. It is not too hard, and if you feel up to the challenge, go ahead.
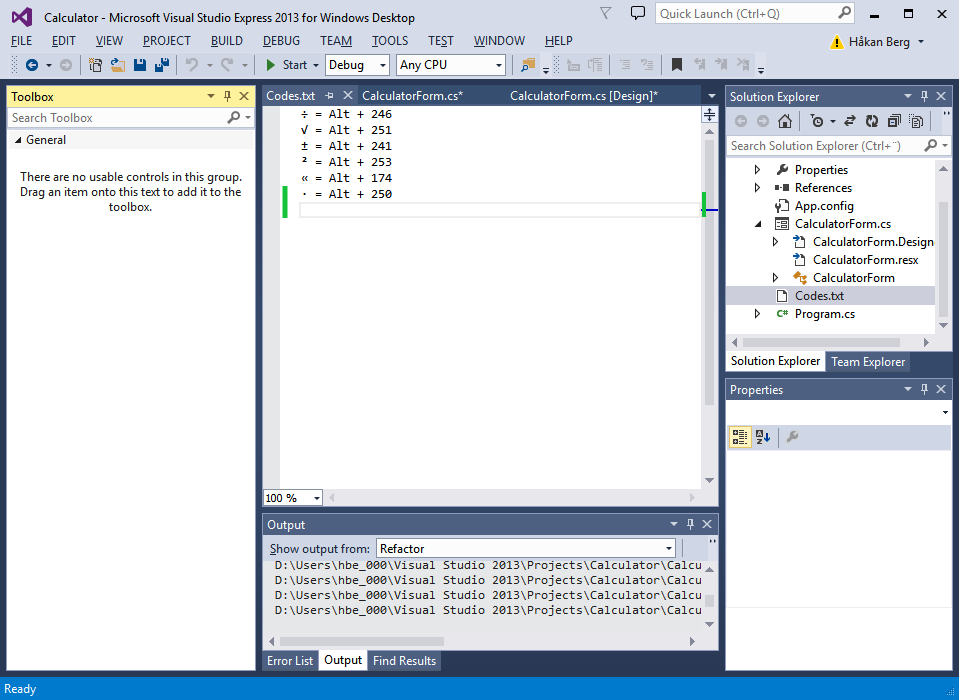
Double-click on each button to create the handler methods. I suggest you do that in such order that buttons that have similar functions are grouped together:
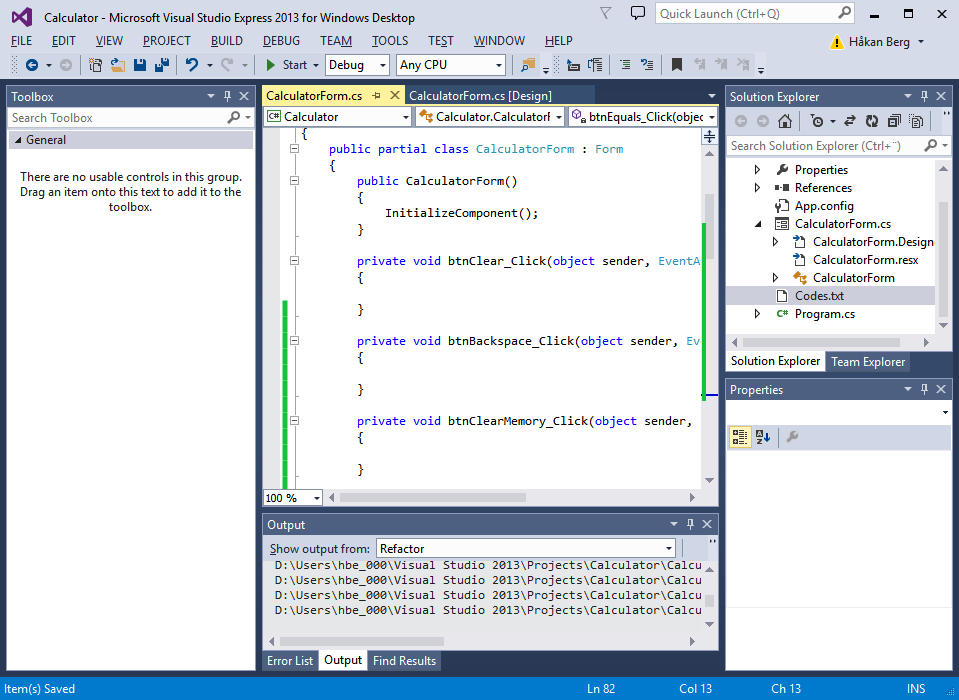
Do not add a handler for the textbox. It is to be used for display only, so better make it read-only. When you are at changing properties, maybe you'd like to make the display right-aligned too:
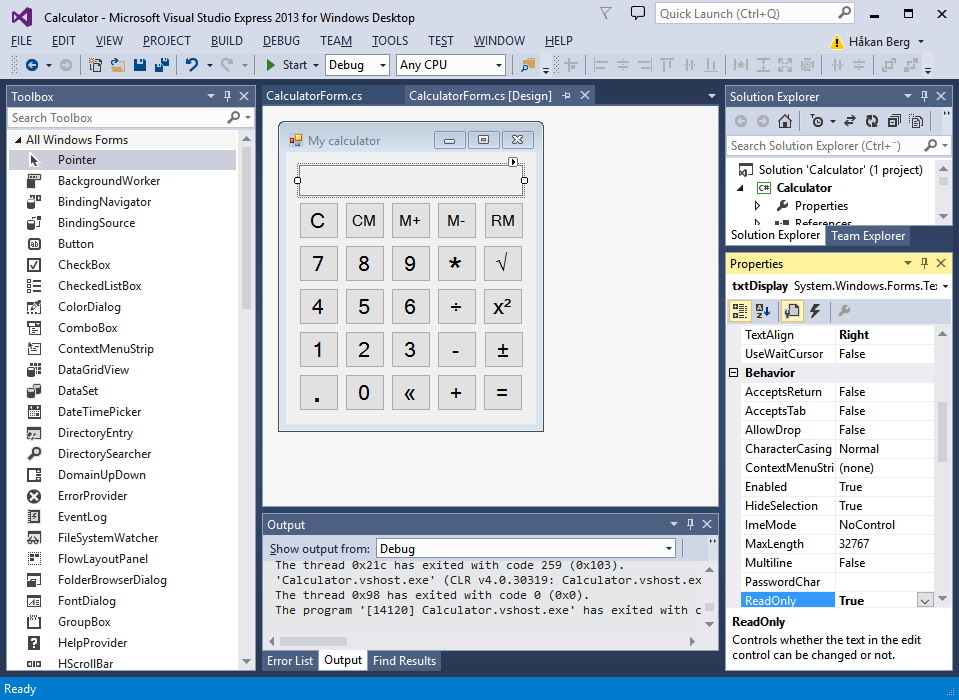
Now comes the tricky part. How to implement the calculator? Think about how you operate a calculator. You enter some digits, click an operator button (+, -, * or /) and then you enter some more digits. When you click the operator button the previously entered digits still remain in the display, but when you start entering the next number, the display is first cleared. Where is the first value stored? It is still needed when you click the equal sign, or next operator.
So, we need two things here. We need somewhere to store the digits we are keying in, a work register, but also somewhere to store the previous result, the current value.
We also have buttons for memory handling, so we must add a variable to hold the memory content.
And since we must remember to clear the display when keying in next number after having clicked an operator button or the equal sign, we need a boolean variable as a flag to control that behaviour.
Finally, it is good practise to create enumerators rather than having an integer to store information about things like the operator keys pressed last. I have declared an enumerator type called '_operation' and a variable 'operation' of that type.
Enter all needed variables at the top of the class since they need to be class global:
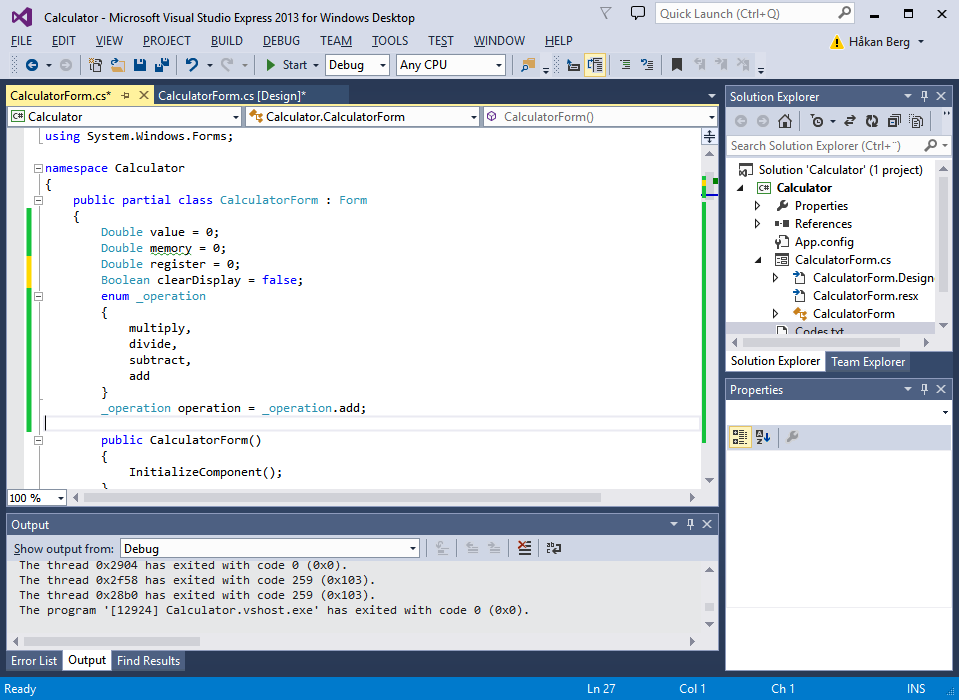
Each time you click a digit button, or the period button, almost the same thing should be done. Rather than repeating very similar code in all those 11 button handlers, it is better to create one function that handles all, and that takes one argument that tells it what button was pressed. Then all hanlers can look similar:
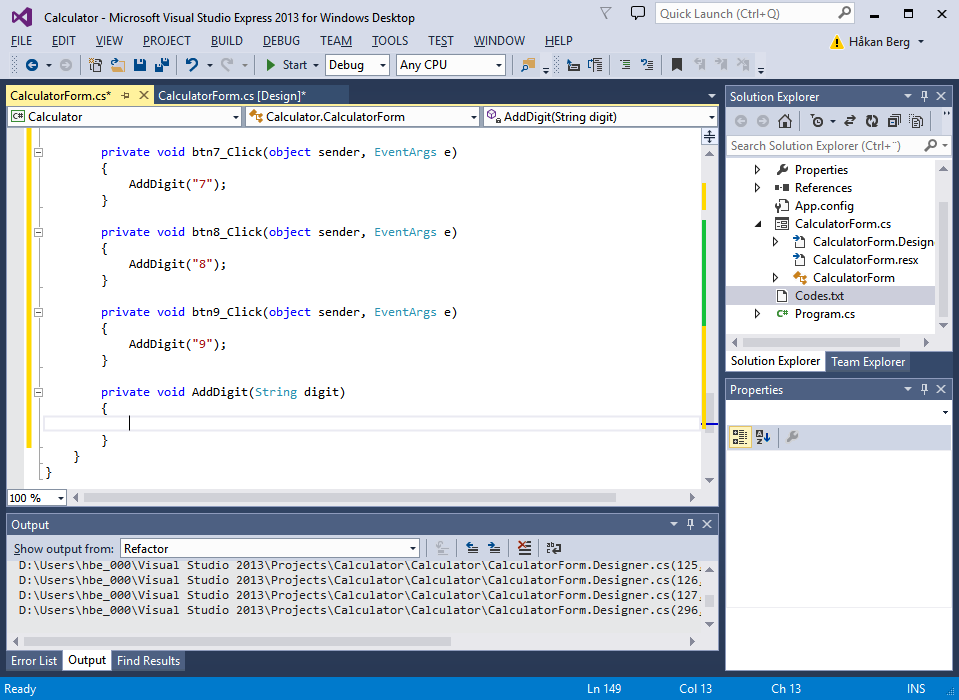
The AddDigit() function has a few things to do:
- Check the boolean to decide whether to clear the display first or not.
- Fetch the current text from the display. (Yes, it is text even if it contains only digits.)
- Bail out if the user tries to enter more than one period. That would be a catastrophy when we later try to convert it to a Double value!
- Add the supplied argument at end of the text.
- Convert the new text to a Double and store it in the work register.
- Update the display.
This is how I did it:
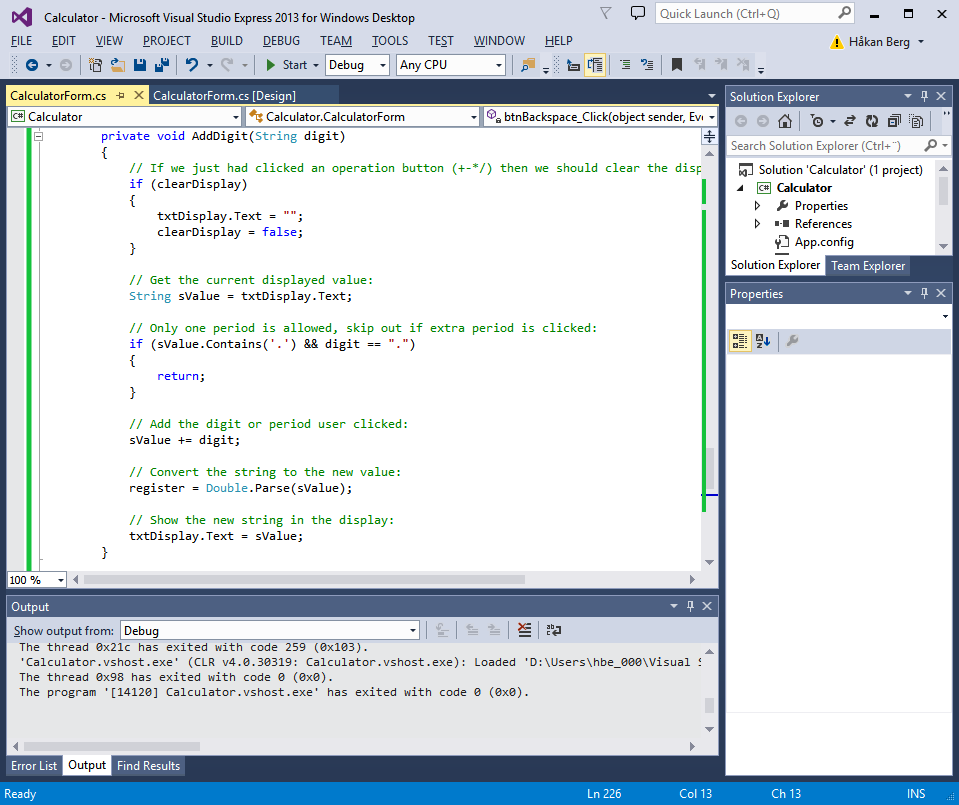
It is now possible to key in digits an one period.
The backspace key can be handled in a similar way. This is what needs to be done:
- Fetch the current text from the display.
- Remove one character from the string.
- Convert the string to Double and store in the work register.
- Update the display.
One may argue that using a local String variable is not nessecary here, nor in AddDigit(), but I did that more for clarity. The syntax becomes slightly clearer, but then again, we get more code. Still, it demonstrates the use of local variables in contrast to the global ones we declared before.
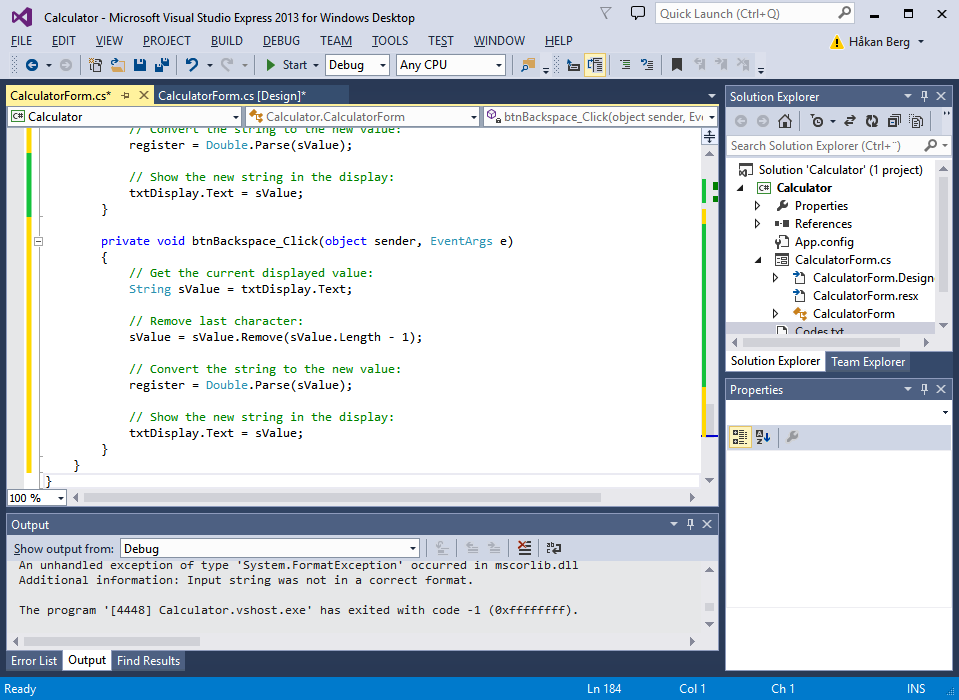
Implement the operator key handlers. It is the same thing here, most is the same, and it is better to write a separate function for the common handling. Here I call it Calculate(). The only thing that differs it what operator is selected:
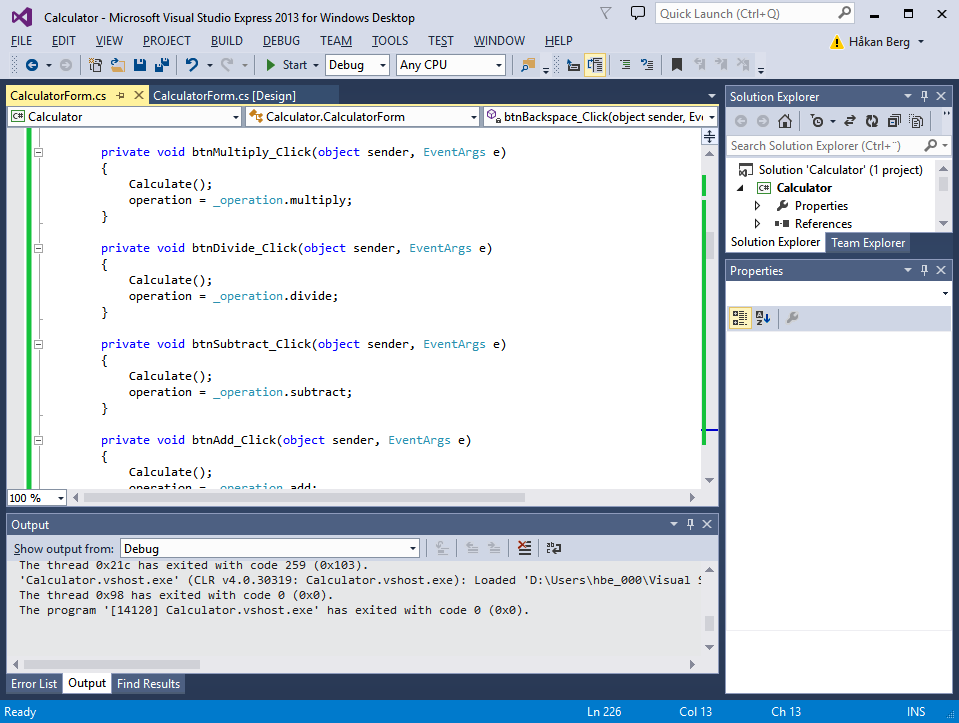
The implementation of Calculate() can be quite simple in this not too advanced calculator.
Depending on whether we already have a value keyed in or not we will either operate on the value and the work register, or store the work register value.
- Depending on the stored value...
- If zero, store the work register value.
- Else, apply the selected operator on the work register to calculate a new value.
- Update the display.
- Reset the work register.
- Set a flag that says that the display should be cleared when keying in the next value.
This is how I implemented it:
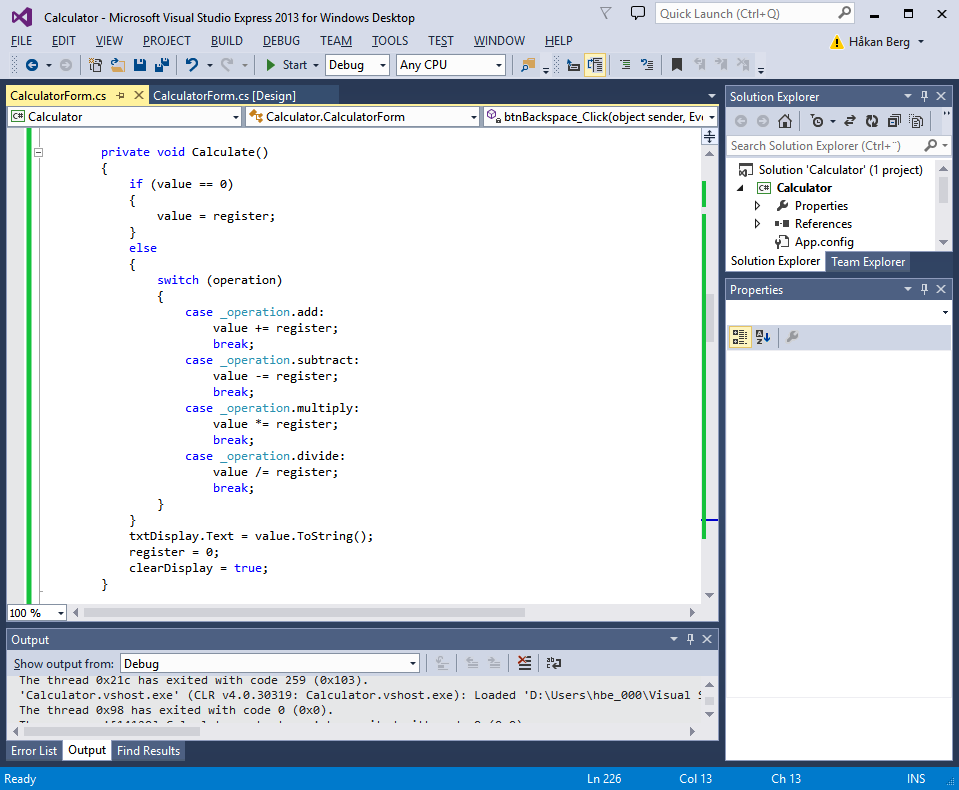
I really should include comments in the code, but since all is explained above, that would just be repeating the same words while cluttering up the code. I hope you insert comments into your code. If you want to change the program six months from now, you might not understand how to do it without comments.
Implementing the memory functions is really simple:
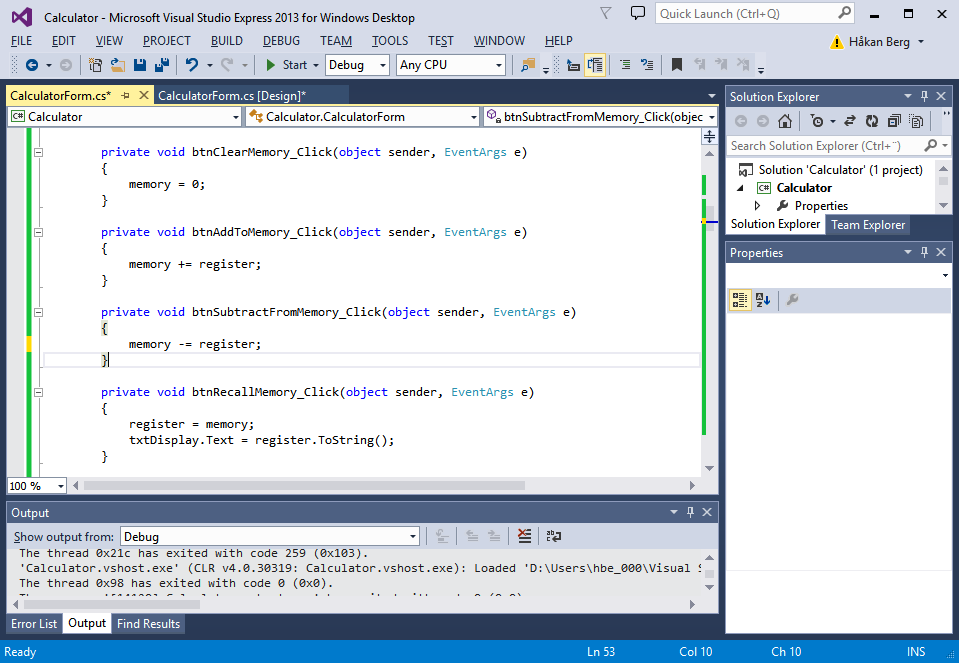
The Math library makes implementing the speial functions easy:
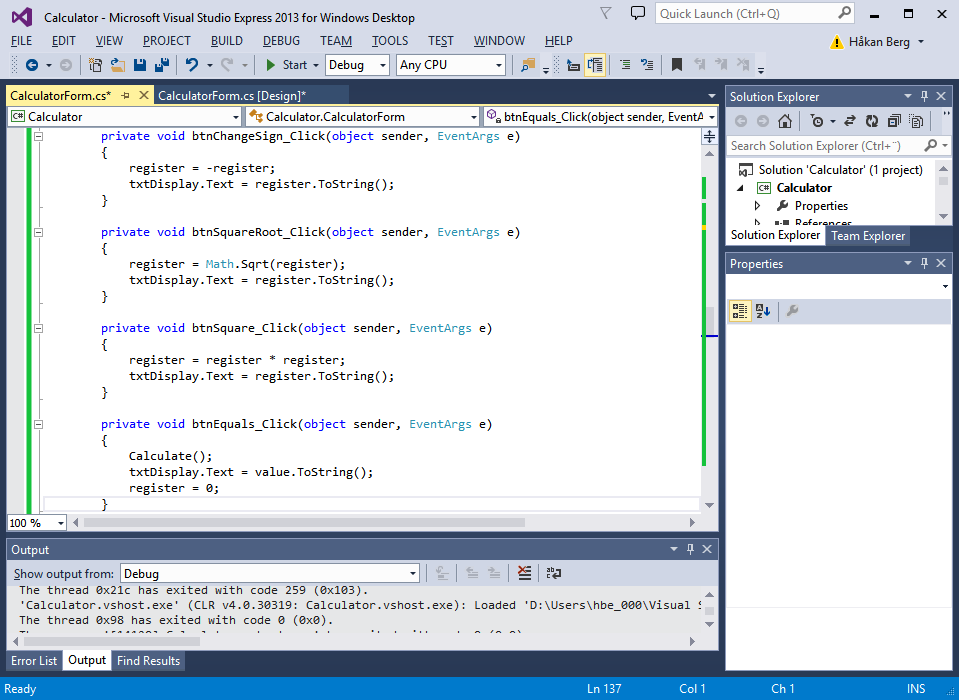
Now, just try it out!
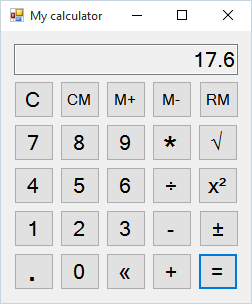
If the calculator did not work properly, set a breakpoint and start debugging.